Spring Integration 4.0: A complete XML-free example
Integration, mongoDB, Spring, Spring WS ·- Introduction.
- An overview of the flow.
- Spring configuration.
- Detail of the endpoints.
- Testing the entire flow.
- Conclusion.
The source code can be found at github.
The source code of the web service invoked in this example can be found at the spring-samples repository at github.
1 An overview of the flow
The example application shows how to configure several messaging and integration endpoints. The user asks for a course by specifying the course Id. The flow will invoke a web service and return the response to the user. Additionally, some type of courses will be stored to a database.
The flow is as follows:
- An integration gateway (course service) serves as the entry to the messaging system.
- A transformer builds the request message from the user specified course Id.
- A web service outbound gateway sends the request to a web service and waits for a response.
- A service activator is subscribed to the response channel in order to return the course name to the user.
- A filter is also subscribed to the response channel. This filter will send some types of courses to a mongodb channel adapter in order to store the response to a database.
The following diagram better shows how the flow is structured:
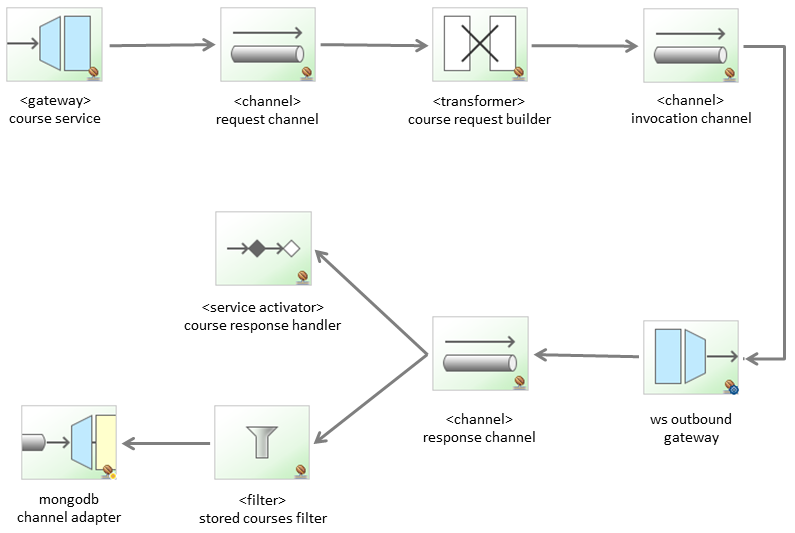
2 Spring configuration
As discussed in the introduction section, the entire configuration is defined with JavaConfig. This configuration is split into three files: infrastructure, web service and database configuration. Let’s check it out:
2.1 Infrastructure configuration
This configuration file only contains the definition of message channels. The messaging endpoints (transformer, filter, etc…) are configured with annotations.
InfrastructureConfiguration.java
@Configuration @ComponentScan("xpadro.spring.integration.endpoint") //@Component @IntegrationComponentScan("xpadro.spring.integration.gateway") //@MessagingGateway @EnableIntegration @Import({MongoDBConfiguration.class, WebServiceConfiguration.class}) public class InfrastructureConfiguration { @Bean @Description("Entry to the messaging system through the gateway.") public MessageChannel requestChannel() { return new DirectChannel(); } @Bean @Description("Sends request messages to the web service outbound gateway") public MessageChannel invocationChannel() { return new DirectChannel(); } @Bean @Description("Sends web service responses to both the client and a database") public MessageChannel responseChannel() { return new PublishSubscribeChannel(); } @Bean @Description("Stores non filtered messages to the database") public MessageChannel storeChannel() { return new DirectChannel(); } }
The @ComponentScan annotation searches for @Component annotated classes, which are our defined messaging endpoints; the filter, the transformer and the service activator.
The @IntegrationComponentScan annotation searches for specific integration annotations. In our example, it will scan the entry gateway which is annotated with @MessagingGateway.
The @EnableIntegration annotation enables integration configuration. For example, method level annotations like @Transformer or @Filter.
2.2 Web service configuration
This configuration file configures the web service outbound gateway and its required marshaller.
WebServiceConfiguration.java
@Configuration public class WebServiceConfiguration { @Bean @ServiceActivator(inputChannel = "invocationChannel") public MessageHandler wsOutboundGateway() { MarshallingWebServiceOutboundGateway gw = new MarshallingWebServiceOutboundGateway("http://localhost:8080/spring-ws-courses/courses", jaxb2Marshaller()); gw.setOutputChannelName("responseChannel"); return gw; } @Bean public Jaxb2Marshaller jaxb2Marshaller() { Jaxb2Marshaller marshaller = new Jaxb2Marshaller(); marshaller.setContextPath("xpadro.spring.integration.ws.types"); return marshaller; } }
The gateway allows us to define its output channel but not the input channel. We need to annotate the adapter with @ServiceActivator in order to subscribe it to the invocation channel and avoid having to autowire it in the message channel bean definition.
2.3 Database configuration
This configuration file defines all necessary beans to set up mongoDB. It also defines the mongoDB outbound channel adapter.
MongoDBConfiguration.java
@Configuration public class MongoDBConfiguration { @Bean public MongoDbFactory mongoDbFactory() throws Exception { return new SimpleMongoDbFactory(new MongoClient(), "si4Db"); } @Bean @ServiceActivator(inputChannel = "storeChannel") public MessageHandler mongodbAdapter() throws Exception { MongoDbStoringMessageHandler adapter = new MongoDbStoringMessageHandler(mongoDbFactory()); adapter.setCollectionNameExpression(new LiteralExpression("courses")); return adapter; } }
Like the web service gateway, we can’t set the input channel to the adapter. I also have done that by specifying the input channel in the @ServiceActivator annotation.
3 Detail of the endpoints
The first endpoint of the flow is the integration gateway, which will put the argument (courseId) into the payload of a message and send it to the request channel.
@MessagingGateway(name = "entryGateway", defaultRequestChannel = "requestChannel") public interface CourseService { public String findCourse(String courseId); }
The message containing the course id will reach the transformer. This endpoint will build the request object that the web service is expecting:
@Component public class CourseRequestBuilder { private Logger logger = LoggerFactory.getLogger(this.getClass()); @Transformer(inputChannel="requestChannel", outputChannel="invocationChannel") public GetCourseRequest buildRequest(Message<String> msg) { logger.info("Building request for course [{}]", msg.getPayload()); GetCourseRequest request = new GetCourseRequest(); request.setCourseId(msg.getPayload()); return request; } }
Subscribed to the response channel, which is the channel where the web service reply will be sent, there’s a service activator that will receive the response message and deliver the course name to the client:
@Component public class CourseResponseHandler { private Logger logger = LoggerFactory.getLogger(this.getClass()); @ServiceActivator(inputChannel="responseChannel") public String getResponse(Message<GetCourseResponse> msg) { GetCourseResponse course = msg.getPayload(); logger.info("Course with ID [{}] received: {}", course.getCourseId(), course.getName()); return course.getName(); } }
Also subscribed to the response channel, a filter will decide based on its type, if the course is required to be stored to a database:
@Component public class StoredCoursesFilter { private Logger logger = LoggerFactory.getLogger(this.getClass()); @Filter(inputChannel="responseChannel", outputChannel="storeChannel") public boolean filterCourse(Message<GetCourseResponse> msg) { if (!msg.getPayload().getCourseId().startsWith("BC-")) { logger.info("Course [{}] filtered. Not a BF course", msg.getPayload().getCourseId()); return false; } logger.info("Course [{}] validated. Storing to database", msg.getPayload().getCourseId()); return true; } }
4 Testing the entire flow
The following client will send two requests; a BC type course request that will be stored to the database and a DF type course that will be finally filtered:
@RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration(classes={InfrastructureConfiguration.class}) public class TestApp { @Autowired CourseService service; @Test public void testFlow() { String courseName = service.findCourse("BC-45"); assertNotNull(courseName); assertEquals("Introduction to Java", courseName); courseName = service.findCourse("DF-21"); assertNotNull(courseName); assertEquals("Functional Programming Principles in Scala", courseName); } }
This will result in the following console output:
5 Conclusion
We have learnt how to set up and test an application powered with Spring Integration using no XML configuration. Stay tuned, because Spring Integration Java DSL with Spring Integration extensions is on its way!
I’m publishing my new posts on Google plus and Twitter. Follow me if you want to be updated with new content.